Basic Robot Movement
Creating a Raspberry PI robot is one of the interesting projects to get started with when attempting to hack together your own DIY projects. The projects tend to have moderate complexity, while giving immense satisfaction when you see the results of your hardwork.
We will need to procure some parts to get started. My preferred destination is Amazon to procure these items.
List of things needed:
- Raspberry Pi
- Rpi camera : X1
- 180 Degree servo motor: X2
- Mini Pan/Tilt Camera Platform Anti-Vibration Camera Mount w/ 2 Servos Aircraft FPV
- LED Red
- Resistor 220 ohms
- Resistor 1K ohm (2X) – Optional
Some additional miscellaneous parts: metal parts, bands, etc (in case you will construct your Pan/Tilt mechanism)
Links to the items purchased from Amazon:
Techleads SG90 + ultrasonic combo kit for arduino | Arduino Project Kit
Nylon FPV Pan/Tilt Camera Mount and SG90 9 G Servo Retail (Set of 2 Pieces)
2 of Kit4Curious NASA Tech Acrylic 2 Wheel Drive Curious Chassis for DIY Robotics (Black)
Raspberry PI 5MP Camera Board Module
Robodo Electronics L298 Motor Driver Module
Jumper Wires Male to Male, male to female, female to female, 120 Pieces
Let us get started with programming our Raspberry Pi robot to do some basic movements.
Step 1
Building the pan tilt camera:
At first, we need to assemble the pan tilt camera mount that we have ordered from our favorite online store. The camera mount usually comes in disassembled form and its helpful to look up some of the online tutorials when doing the assembly.
The following video has very useful steps:
Pan and Tilt Sensor Mount Assembly for Arduino and Raspberry Pi
Make sure you stop before completing the last step. Assemble both the parts and then screw in the tilt motor screws. If not, then they will not go in post full assembly.

Step 2
Assembling the chassis:
Robot chassis assembly: https://www.youtube.com/watch?v=mLc6mbt7maw
Ultrasonic sensor assembly: https://www.youtube.com/watch?v=FO_mJcxquqk
Step 3:
It is always a good practice to review the datasheet of key components like the L298 H-bridge driver. The familiarity will help avoid any inadvarent mistakes that can potentially cause damage to the components. Data sheet of L298 H-bridge motor driver:
https://cdn.instructables.com/ORIG/FCN/YABW/IHNTEND4/FCNYABWIHNTEND4.pdf
Step 4:
Creating a Raspbian based image for programming the Raspberry Pi robot. My Raspberry Pi is a headless unit, so I would be programming the wifi details offline with a static IP, so that the device comes up with pre-configured wi-fi network.
- Flashing latest version of Raspbian on uSD card using etcher
- Create a file called wpa_supplicant.conf in /boot of uSD card
- This will get copied over to the main filesystem on first boot
ctrl_interface=DIR=/var/run/wpa_supplicant GROUP=netdev
update_config=1
country=IN
network={
ssid="xxxxxx"
psk="xxxxxxxx"
key_mgmt=WPA-PSK
}
- Create a empty file called ssh to enable SSH on first boot
- USB -C issue:
- Assigning a static IP
- Boot the PI
- Login to router and determine DHCP IP. In my case, the PI got the DHCP IP 192.168.1.9
- Login using ssh pi@192.168.1.9
- Password: raspberry
- Enable root access from SSH
- login as a root user
- pi@raspberrypi:~ $ sudo -i
- see the user name ‘root’
- root@raspberrypi:~#
- set a new password for the root user
- root@raspberrypi:~# passwd
#Enter
new UNIX password:
#Retype new UNIX password:
#passwd: password updated successfully
root@raspberrypi:~#
To access pi as a ‘Root’ user via ssh, we need to allow ‘PermitRootLogin’.
#set
‘PermitRootLogin’ as ‘yes’
root@raspberrypi:~# nano /etc/ssh/sshd_config
#Find
‘PermitRootLogin prohibit-password’ text and replace it as ‘PermitRootLogin
yes’
PermitRootLogin yes
#save sshd_config file (ctrl + x)
#3.
Enter Following command to create a backup file
root@raspberrypi:~# cp /etc/dhcpcd.conf /etc/dhcpcd.conf.backup
#4.
Open dhcpcd.conf file
root@raspberrypi:~# nano /etc/dhcpcd.conf
#Based
on the above information (#1 and #2), we will add following lines to the top of
dhcpcd.conf
# We want to use static ip_address as 192.168.179.111 (based on #1, it should
be in between lower address bound 192.168.179.1 and upper address bound 192.168.179.255),
values of static routers and static domain_name_servers (based on #2)
interface
wlan0
static ip_address=192.168.179.111
static routers=192.168.179.1
static domain_name_servers=192.168.179.1
#save
the file (Ctrl + x) and reboot your pi
root@raspberrypi:~# reboot
- Your PI should be up with the new IP
- Set up the board/motor as per the below schematic. Make sure that the ground is common between H298, power supply and RPI. This is a really important step and really sent me down a wild goose chase.
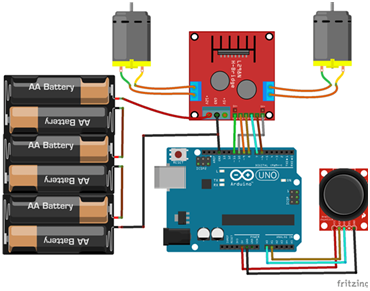
- On the PI side, install the latest wiringpi application. This will provide the GPIO app.
- Type: gpio readall
- To see all the GPIOs.
- Run the following code. (from: https://projects.raspberrypi.org/en/projects/build-a-buggy/3 )
Debugging H298:
- To ensure that H298 is fine, use the 5V out pin and touch the wire to INPUT 1/2/3/4. The motors on each side should spin and the direction should reverse. If this is working, then your H298 is correctly configured.
Debugging the PI GPIOs:
- Easiest way to confirm the PI GPIOs is to connect a LED and test it out. Connect the -ve to GND PIN in the GPIO header of PI. Connect the +ve to +3.3V and +5V pin

TIP: when programming, the python Robot libraries use the Broadcom numbering (example GPIO17, GPIO27 etc)
The wiring GPIO app uses the pin number in circle.
For my project:
robby = Robot(left=(6,13), right=(26,19))
TIP: Through out this series, we will be extensively using packages and APIs provided by gpiozero package on raspbian. This has really helpful API’s covering most of the standard raspberry PI devices. The excellent documentation is located here: https://gpiozero.readthedocs.io/en/stable/index.html
TIP: Do not use a power bank to supply power to RPI. They cannot meet the current requirements and I bricked by RPI4 trying this.
Debugging that PI is a different post.
NOTE:
Configure default behaviour of GPIO in /boot/config.txt using GPIO directive.
Numbering used is BCM
https://www.raspberrypi.org/documentation/configuration/config-txt/gpio.md
All the code is available in Bitbucket. Use the following command to download the repo:
git clone https://boseontherocks@bitbucket.org/boseontherocks/projects_1.git
The code used in this post is available in the folder robot_1